-
Notifications
You must be signed in to change notification settings - Fork 31
Compute Flattened State Transition Map
This algorithm flattens the state transitions in a state machine definition. By flattening transitions, we simplify the code generation and runtime logic.
-
A state machine definition smd.
-
A state machine analysis data structure sma representing the results of analysis so far.
-
sma with the flattened state transition map updated.
The following diagrams illustrate how state transitions are flattened.
Figure 1 shows how transitions are flattened where, after the flattening, the target state T is not a transitive parent of the source state S (i.e., T is not S, or a parent of S, or a parent of a parent of S, etc.). In this example, S1 is a state with substates S2 and S3. S4 is a state with substate S5.
The solid left-to-right arrow represents a transition T in the hierarchical state machine from S1 to S5 with actions A. T generates each of the flattened transitions represented by the dashed arrows. Neither of the arrows goes from a state S to a transitive parent of S. For each dashed arrow, the action list is the concatenation of the action lists shown. In these lists, exit(S) represents the list of exit functions specified by S, and similarly for entry(S).
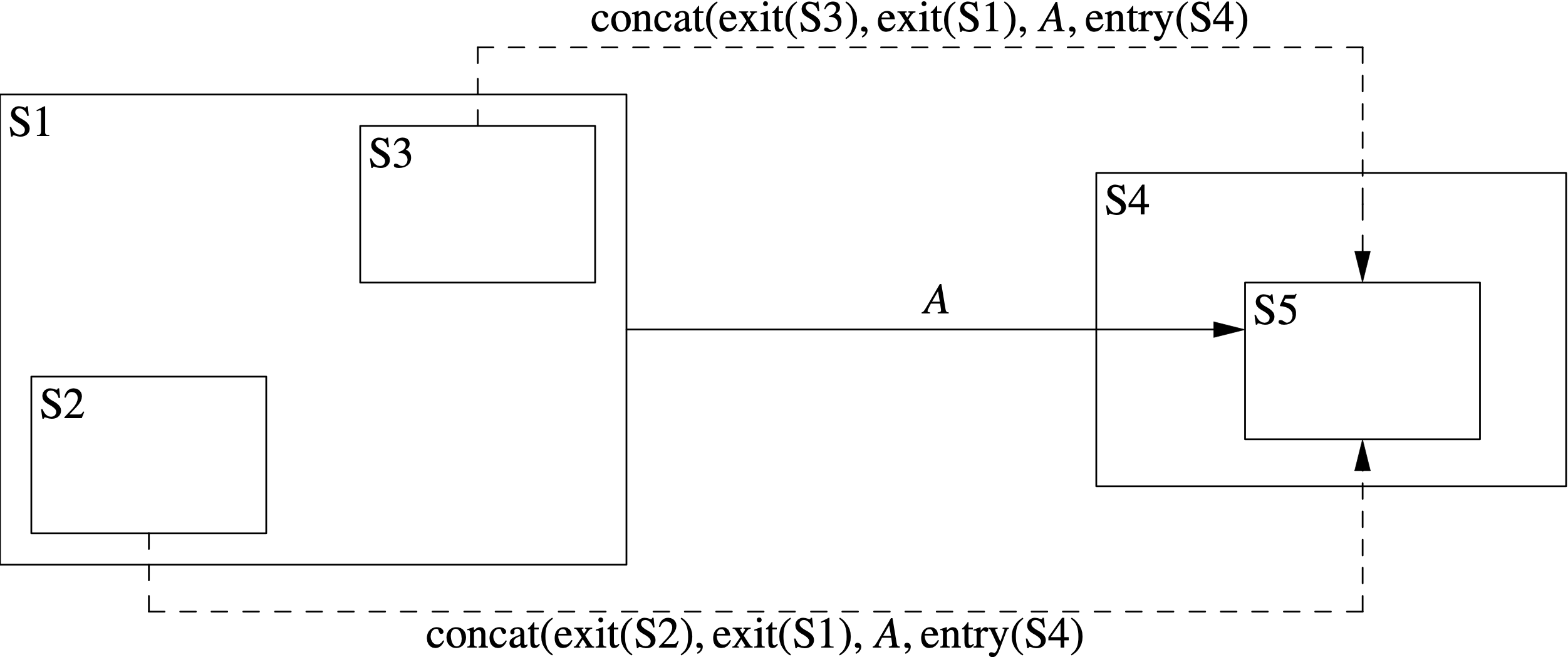
Note the following:
-
Each flattened transition goes from a leaf state but not necessarily to a leaf state.
-
In general, T generates the transitions out of S2 and S3 as shown. However, if the hierarchical state machine specifies a transition T' out of S2, and T' is triggered by the same signal as T, then T' overrides T; and similarly for a transition T'' out of S3. This rule is called behavioral polymorphism.
Figure 2 shows how transitions are flattened where, after the flattening, the transition goes from a state S to a transitive parent of S. Again S1 is a state with substates S2 and S3.
The solid clockwise arrow represents a transition T in the hierarchical state machine from S1 to itself with actions A. T generates each of the flattened transitions represented by the dashed arrows. Each arrow goes from a state S to a transitive parent of S. For each dashed arrow, the action list is the concatenation of the action lists shown.

Note the following:
-
When a flattened transition goes from a state S to a transitive parent P of S, we exit and re-enter P. For purposes of this rule, S is a transitive parent of itself.
-
Again the transition out of S2 will be be overridden if there is a transition specified in S2 on the same signal, and similarly for S3.
Figure 3 shows how state transitions to choices are flattened. Again S1 is a state with substates S2 and S3. S4 is a state with a choice definition C. The solid left-to-right arrow represents a transition T in the hierarchical state machine from S1 to C with actions A. T generates each of the flattened transitions represented by the dashed arrows.
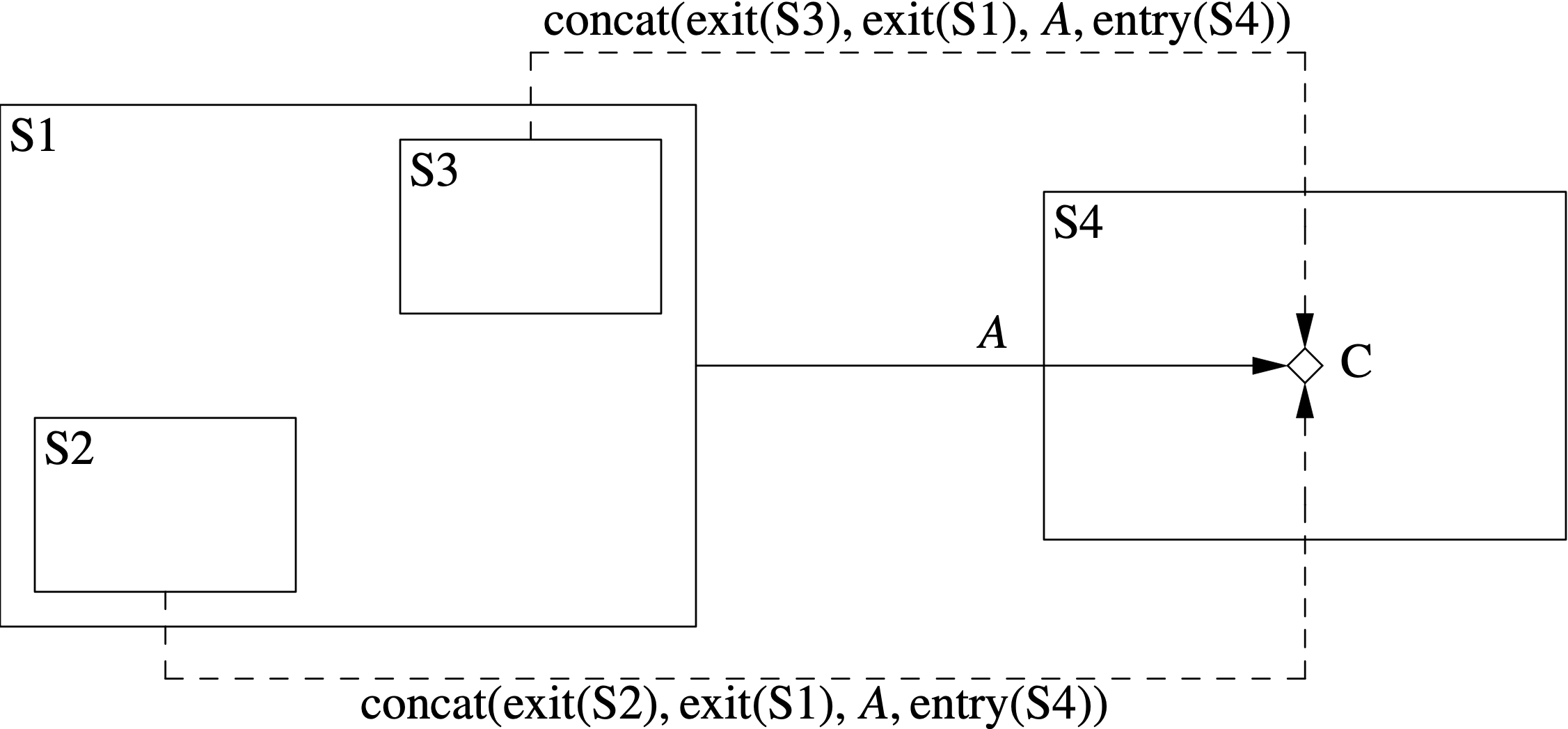
Note the following:
-
Each flattened transition goes from a leaf state to a choice.
-
Choices do not have entry actions or substates.
-
Again the transition out of S2 will be be overridden if there is a transition specified in S2 on the same signal, and similarly for S3.
Figure 4 shows how internal state transitions are flattened. Again S1 is a state with substates S2 and S3.
The solid arrow represents an internal transition T in the hierarchical state machine from S1 to itself with actions A. T generates each of the flattened internal transitions represented by the dashed arrows.
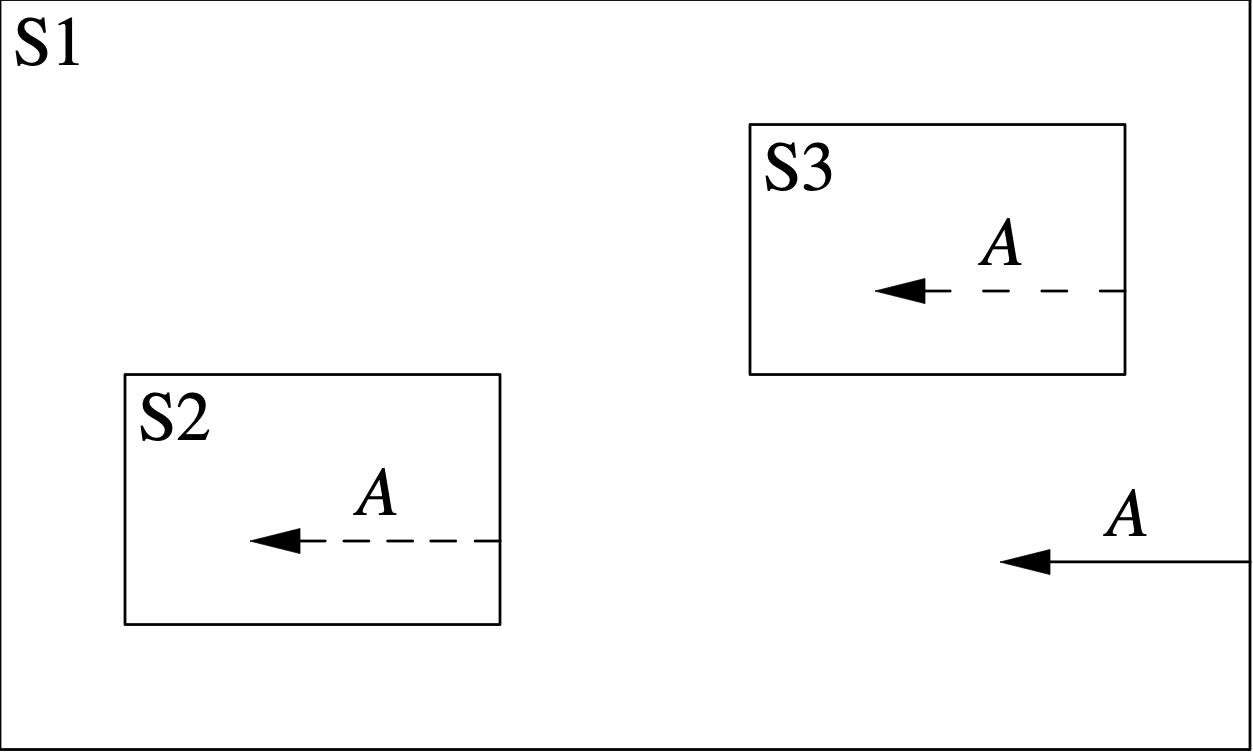
Note the following:
-
When flattening an internal transition, we do exit or enter any states.
-
Again the transition from S2 will be be overridden if there is a transition specified in S2 on the same signal, and similarly for S3.
-
Let stm be the signal-transition-map of sma.
-
Let fstm be the flattened state transition map of sma.
-
Set each of stm and fstm to the empty map.
-
Visit each state definition that is a direct member of smd.
-
Let sd be the state definition being visited.
-
Let stm' = stm.
-
For each state transition specifier sts in sd
-
Let s be the signal of sts, let g be the optional guard of sts, and let A be the actions of sts.
-
If sts specifies an internal transition (i.e., sts has actions but no target), then let t be the internal transition with actions A.
-
Otherwise let t be the external transition whose actions are A and whose target is the target of sts.
-
Update stm so that it maps s to (g,t). If any preexisting transition is mapped to s, it is overridden. Because we walk the AST in depth-first order, a transition specified lower in the tree overrides any transition on the same signal specified higher in the tree. Thus the algorithm respects behavioral polymorphism.
-
-
If sd is a leaf state, then
-
For each signal s in stm
-
Let (g,t) = stm(s).
-
Construct the flattened transition t' with source sd and transition t.
-
If fstm(s) does not exist, then set fstm(s) to the empty map.
-
Add the mapping from sd to (g,t') to fstm(s).
-
-
-
Otherwise for each child state definition sd' of sd, visit sd'.
-
Set stm = stm'.